新建
PyTorch Tutorial | 1.Tensor
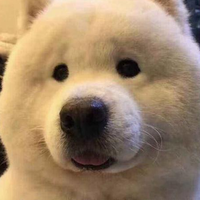
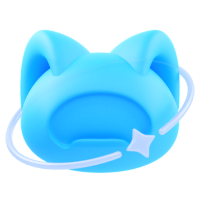
更新于 2024-12-14
推荐镜像 :Basic Image:ubuntu:22.04-py3.10-pytorch2.0
推荐机型 :c2_m4_cpu
赞
目录
[82]
# import packages
import torch
import numpy as onp
代码
文本
Initializing a tensor
代码
文本
[83]
# create tensor object from list & numpy array
data = [[1,2],[3,4]]
x_data = torch.tensor(data)
x_np = torch.from_numpy(onp.array(data))
代码
文本
[84]
# from another tensor
x_ones = torch.ones_like(x_data)
print(f"Ones Tensor:\n{x_ones}")
x_rand = torch.rand_like(x_data, dtype = torch.float)
print(f"Random Tensor:\n{x_rand}")
Ones Tensor: tensor([[1, 1], [1, 1]]) Random Tensor: tensor([[0.4822, 0.5874], [0.2995, 0.2137]])
代码
文本
[85]
# with shape paramters
shape = (2,3)
rand_tensor = torch.rand(shape)
print(f"Random Tensor:\n{rand_tensor}")
ones_tensor = torch.ones(shape)
print(f"Ones Tensor:\n{ones_tensor}")
zeros_tensor = torch.zeros(shape)
print(f"Zeros Tensor:\n{zeros_tensor}")
Random Tensor: tensor([[0.5966, 0.2829, 0.1161], [0.9587, 0.7492, 0.6312]]) Ones Tensor: tensor([[1., 1., 1.], [1., 1., 1.]]) Zeros Tensor: tensor([[0., 0., 0.], [0., 0., 0.]])
代码
文本
Attributes of a Tensor
代码
文本
[86]
tensor = torch.rand(4,3,4)
print(f"Shape: {tensor.shape}")
print(f"Size: {tensor.size()}")
print(f"Dtype: {tensor.dtype}")
print(f"Devices: {tensor.device}")
Shape: torch.Size([4, 3, 4]) Size: torch.Size([4, 3, 4]) Dtype: torch.float32 Devices: cpu
代码
文本
Operations on Tensors
代码
文本
[87]
'''
By default, Tensors are created on CPU
We need to explicitly move them to GPU
'''
# Move large tensors could be expensive!
tensor = torch.rand(100,100,100)
if torch.cuda.is_available():
tensor = tensor.to("cuda")
print(f"Move to GPU")
else:
print(f"Only CPU")
Only CPU
代码
文本
[88]
# PyTorch has similar tensor related API as Numpy
tensor = torch.rand(3,3)
print(tensor)
print(f"First row: {tensor[0,:]}")
print(f"First column: {tensor[:,0]}")
print(f"Last column: {tensor[:,-1]}")
tensor[:,0] = 1
print(tensor)
tensor([[0.9287, 0.2697, 0.6956], [0.0174, 0.1157, 0.9993], [0.6828, 0.8940, 0.0911]]) First row: tensor([0.9287, 0.2697, 0.6956]) First column: tensor([0.9287, 0.0174, 0.6828]) Last column: tensor([0.6956, 0.9993, 0.0911]) tensor([[1.0000, 0.2697, 0.6956], [1.0000, 0.1157, 0.9993], [1.0000, 0.8940, 0.0911]])
代码
文本
[89]
# joining tensors
t1 = torch.cat([tensor, tensor, tensor], dim=1)
print(t1)
tensor([[1.0000, 0.2697, 0.6956, 1.0000, 0.2697, 0.6956, 1.0000, 0.2697, 0.6956], [1.0000, 0.1157, 0.9993, 1.0000, 0.1157, 0.9993, 1.0000, 0.1157, 0.9993], [1.0000, 0.8940, 0.0911, 1.0000, 0.8940, 0.0911, 1.0000, 0.8940, 0.0911]])
代码
文本
[90]
# matrix multiplication (following three ways are the equavalient)
tensor = torch.tensor([[1.,2.],[3.,4.]])
y1 = tensor @ tensor.T
y2 = torch.matmul(tensor, tensor.T)
y3 = torch.rand_like(y1)
torch.matmul(tensor, tensor.T, out=y3)
for i, y in enumerate([y1,y2,y3]):
print(f"y{i+1}:\n{y}")
y1: tensor([[ 5., 11.], [11., 25.]]) y2: tensor([[ 5., 11.], [11., 25.]]) y3: tensor([[ 5., 11.], [11., 25.]])
代码
文本
[91]
# element-wise product (also same)
z1 = tensor * tensor
z2 = tensor.mul(tensor)
z3 = torch.rand_like(z1)
torch.mul(tensor, tensor, out=z3)
for i, z in enumerate([z1,z2,z3]):
print(f"z{i+1}:\n{z}")
z1: tensor([[ 1., 4.], [ 9., 16.]]) z2: tensor([[ 1., 4.], [ 9., 16.]]) z3: tensor([[ 1., 4.], [ 9., 16.]])
代码
文本
[92]
# signle-element tenor
agg = tensor.sum()
agg_item = agg.item()
print(agg_item, type(agg_item))
10.0 <class 'float'>
代码
文本
[93]
'''
in-place oeprations (change the operand), with "_" suffix
'''
print(tensor)
tensor.add_(10)
print(tensor)
tensor([[1., 2.], [3., 4.]]) tensor([[11., 12.], [13., 14.]])
代码
文本
Bridge with Numpy
代码
文本
[98]
# Tensor to Numpy array
t = torch.ones(3,3)
print(f"t:\n{t}")
n = t.numpy()
print(f"n:\n{n}")
t: tensor([[1., 1., 1.], [1., 1., 1.], [1., 1., 1.]]) n: [[1. 1. 1.] [1. 1. 1.] [1. 1. 1.]]
代码
文本
[108]
# Numpy array to tensor
n = onp.ones(5)
t = torch.from_numpy(n)
print((n))
print(t)
# changes in NumPy array reflects in the tensor
n[1] = 2
print((n))
print(t)
[1. 1. 1. 1. 1.] tensor([1., 1., 1., 1., 1.], dtype=torch.float64) [1. 2. 1. 1. 1.] tensor([1., 2., 1., 1., 1.], dtype=torch.float64)
代码
文本
点个赞吧
本文被以下合集收录
PyTorch Tutorial
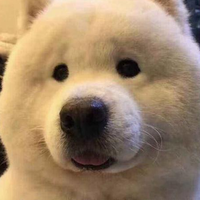
Weipeng Xu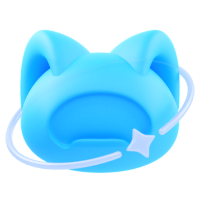
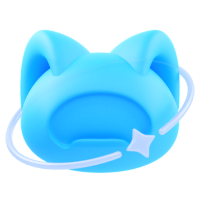
更新于 2024-12-14
2 篇0 人关注