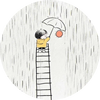
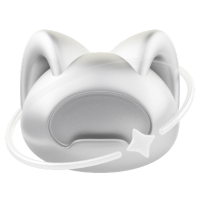
Homework 1: COVID-19 Cases Prediction (Regression)
Objectives:
- Solve a regression problem with deep neural networks (DNN).
- Understand basic DNN training tips.
- Familiarize yourself with PyTorch.
If you have any questions, please contact the TAs via TA hours, NTU COOL, or email to mlta-2023-spring@googlegroups.com
Download data
If the Google Drive links below do not work, you can use the dropbox link below or download data from Kaggle, and upload data manually to the workspace.
Import packages
Some Utility Functions
You do not need to modify this part.
Dataset
Neural Network Model
Try out different model architectures by modifying the class below.
Feature Selection
Choose features you deem useful by modifying the function below.
Training Loop
Configurations
config
contains hyper-parameters for training and the path to save your model.
Dataloader
Read data from files and set up training, validation, and testing sets. You do not need to modify this part.
Start training!
Testing
The predictions of your model on testing set will be stored at pred.csv
.
Download
Run this block to download the pred.csv
by clicking.
Reference
This notebook uses code written by Heng-Jui Chang @ NTUEE (https://github.com/ga642381/ML2021-Spring/blob/main/HW01/HW01.ipynb)
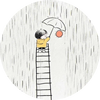
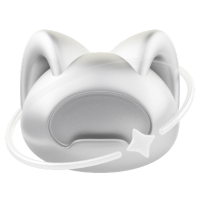
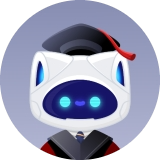
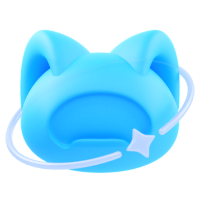
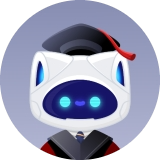
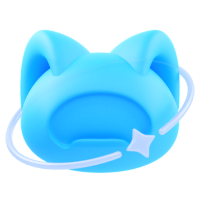