新建
CrySTINet - 基于XRD的晶体结构分类
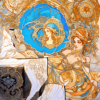
JiaweiMiao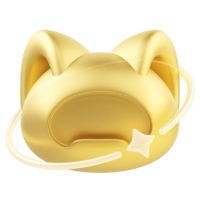
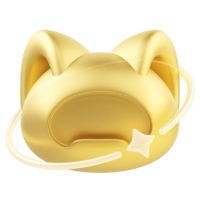
推荐镜像 :Basic Image:bohrium-notebook:2023-03-26
推荐机型 :c12_m92_1 * NVIDIA V100
赞
目录
数据集
CrySTINet(v1)
Crystal Structure Type Recognition Network (CrySTINet)
本Notebook中代码和数据来源:github/PKUsam2023/CrySTINet
代码
文本
Abstract
从实验表征中确定以前未见过的化合物的结构是材料科学的重要组成部分。 它需要一个搜索符合未知化合物晶格的结构类型的步骤,这使得能够对表征数据(例如X射线衍射(XRD)图案)进行图案匹配过程。 然而,这个过程通常对领域专业知识提出很高的要求,从而为计算机驱动的自动化制造了障碍。 在这里,我们通过利用由卷积残差神经网络联合组成的深度学习模型来解决这一挑战。 该模型的准确性在包含 100 种结构类型的 60,000 多种不同化合物的数据集上得到了证明,并且可以集成其他类别,而无需重新训练现有网络。 我们还揭示了深度学习的黑盒操作,并强调了根据 XRD 图案中的局部和全局特征量化未知化合物和结构类型之间的相似性的方式。 这种计算工具为高通量实验发现材料的自动结构分析开辟了新途径。
本问主要内容为单个CrySTINet模型的代码实现以及在测试集上的结果展示。 完整运行代码只需要几分钟。
代码
文本
设置环境
代码
文本
[1]
%%capture
! pip install -r /bohr/crystinet-s17f/v1/CrySTINet/requirements.txt
代码
文本
[2]
import sys
import os
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.utils.data import DataLoader, Dataset
import numpy as np
import pandas as pd
from tqdm import tqdm
代码
文本
CrySTINet 模型
代码
文本
Conv1dPadSame module
代码
文本
[3]
class Conv1dPadSame(nn.Module):
"""
extend nn.Conv1d to support SAME padding
"""
def __init__(self, in_channels, out_channels, kernel_size, stride, groups=1):
super().__init__()
self.in_channels = in_channels
self.out_channels = out_channels
self.kernel_size = kernel_size
self.stride = stride
self.groups = groups
self.conv = torch.nn.Conv1d(
in_channels=self.in_channels,
out_channels=self.out_channels,
kernel_size=self.kernel_size,
stride=self.stride,
groups=self.groups)
def forward(self, x):
net = x
in_dim = net.shape[-1]
out_dim = (in_dim + self.stride - 1) // self.stride
p = max(0, (out_dim - 1) * self.stride + self.kernel_size - in_dim)
pad_left = p // 2
pad_right = p - pad_left
net = F.pad(net, (pad_left, pad_right), "constant", 0)
net = self.conv(net)
return net
代码
文本
MaxPool1dPadSame module
代码
文本
[4]
class MaxPool1dPadSame(nn.Module):
"""
extend nn.MaxPool1d to support SAME padding
"""
def __init__(self, kernel_size):
super(MaxPool1dPadSame, self).__init__()
self.kernel_size = kernel_size
self.stride = 1
self.max_pool = torch.nn.MaxPool1d(kernel_size=self.kernel_size)
def forward(self, x):
net = x
in_dim = net.shape[-1]
out_dim = (in_dim + self.stride - 1) // self.stride
p = max(0, (out_dim - 1) * self.stride + self.kernel_size - in_dim)
pad_left = p // 2
pad_right = p - pad_left
net = F.pad(net, (pad_left, pad_right), "constant", 0)
net = self.max_pool(net)
return net
代码
文本
ResNet Basic Block
代码
文本
[5]
class BasicBlock(nn.Module):
"""
ResNet Basic Block
"""
def __init__(self, in_channels, out_channels, kernel_size,
stride, groups, downsample,
use_bn, use_do, is_first_block=False):
super(BasicBlock, self).__init__()
self.in_channels = in_channels
self.kernel_size = kernel_size
self.out_channels = out_channels
self.stride = stride
self.groups = groups
self.downsample = downsample
if self.downsample:
self.stride = stride
else:
self.stride = 1
self.is_first_block = is_first_block
self.use_bn = use_bn
self.use_do = use_do
# the first conv
self.bn1 = nn.BatchNorm1d(in_channels)
self.relu1 = nn.ReLU()
self.do1 = nn.Dropout(p=0.5)
self.conv1 = Conv1dPadSame(
in_channels=in_channels,
out_channels=out_channels,
kernel_size=kernel_size,
stride=self.stride,
groups=self.groups)
# the second conv
self.bn2 = nn.BatchNorm1d(out_channels)
self.relu2 = nn.ReLU()
self.do2 = nn.Dropout(p=0.5)
self.conv2 = Conv1dPadSame(
in_channels=out_channels,
out_channels=out_channels,
kernel_size=kernel_size,
stride=1,
groups=self.groups)
self.max_pool = MaxPool1dPadSame(kernel_size=self.stride)
def forward(self, x):
identity = x
# the first conv
out = x
if not self.is_first_block:
if self.use_bn:
out = self.bn1(out)
out = self.relu1(out)
if self.use_do:
out = self.do1(out)
out = self.conv1(out)
# the second conv
if self.use_bn:
out = self.bn2(out)
out = self.relu2(out)
if self.use_do:
out = self.do2(out)
out = self.conv2(out)
# if downsample, also downsample identity
if self.downsample:
identity = self.max_pool(identity)
# if expand channel, also pad zeros to identity
if self.out_channels != self.in_channels:
identity = identity.transpose(-1,-2)
ch1 = (self.out_channels-self.in_channels)//2
ch2 = self.out_channels-self.in_channels-ch1
identity = F.pad(identity, (ch1, ch2), "constant", 0)
identity = identity.transpose(-1,-2)
# shortcut
out += identity
return out
代码
文本
Res-Confidence Network
代码
文本
[6]
class RCNet(nn.Module):
"""
Res-Confidence Network
Input:
X: (n_samples, n_channel, n_length)
Y: (n_samples)
Output:
out: (n_samples)
Pararmetes:
in_channels: dim of input, the same as n_channel
base_filters: number of filters in the first several Conv layer, it will double at every 4 layers
kernel_size: width of kernel
stride: stride of kernel moving
groups: set larget to 1 as ResNeXt
n_block: number of blocks
n_classes: number of classes
"""
def __init__(self,
in_channels=1,
base_filters=10,
kernel_size=30,
stride=2,
groups=1,
n_block=16,
n_classes=10,
downsample_gap=2,
increasefilter_gap=4,
use_bn=True,
use_do=True):
super().__init__()
self.n_block = n_block
self.kernel_size = kernel_size
self.stride = stride
self.groups = groups
self.use_bn = use_bn
self.use_do = use_do
self.downsample_gap = downsample_gap # 2 for base model
self.increasefilter_gap = increasefilter_gap # 4 for base model
# first block
self.first_block_conv = Conv1dPadSame(in_channels=in_channels,
out_channels=base_filters,
kernel_size=self.kernel_size,
stride=1)
self.first_block_bn = nn.BatchNorm1d(base_filters)
self.first_block_relu = nn.ReLU()
out_channels = base_filters
# residual blocks
self.basicblock_list = nn.ModuleList()
for i_block in range(self.n_block):
# is_first_block
if i_block == 0:
is_first_block = True
else:
is_first_block = False
# downsample at every self.downsample_gap blocks
if i_block % self.downsample_gap == 1:
downsample = True
else:
downsample = False
# in_channels and out_channels
if is_first_block:
in_channels = base_filters
out_channels = in_channels
else:
# increase filters at every self.increasefilter_gap blocks
in_channels = int(base_filters*2**((i_block-1)//self.increasefilter_gap))
if (i_block % self.increasefilter_gap == 0) and (i_block != 0):
out_channels = in_channels * 2
else:
out_channels = in_channels
tmp_block = BasicBlock(
in_channels=in_channels,
out_channels=out_channels,
kernel_size=self.kernel_size,
stride = self.stride,
groups = self.groups,
downsample=downsample,
use_bn = self.use_bn,
use_do = self.use_do,
is_first_block=is_first_block)
self.basicblock_list.append(tmp_block)
# final prediction
self.final_bn = nn.BatchNorm1d(out_channels)
self.final_relu = nn.ReLU(inplace=True)
# self.do = nn.Dropout(p=0.5)
self.dense = nn.Linear(out_channels, n_classes)
self.dense2 = nn.Linear(out_channels, 1)
self.softmax = nn.Softmax(dim=1)
def forward(self, x):
out = x
# first conv
out = self.first_block_conv(out)
if self.use_bn:
out = self.first_block_bn(out)
out = self.first_block_relu(out)
# residual blocks, every block has two conv
for i_block in range(self.n_block):
net = self.basicblock_list[i_block]
out = net(out)
# final prediction
if self.use_bn:
out = self.final_bn(out)
out = self.final_relu(out)
out = out.mean(-1)
# out = self.do(out)
c = torch.sigmoid(self.dense2(out))
out = self.dense(out)
out = self.softmax(out)
return out, c
代码
文本
使用所有模型测试 txt 文件中的单个 xrd 模式
代码
文本
[7]
class CrySTINet:
"""
Test a single xrd pattern from txt file with all models
"""
def __init__(self, model_kind_dict, alpha = 0.7) -> None:
self.model_kind_dict = model_kind_dict
self.alpha = alpha
self.avg_xrd = np.load('/bohr/crystinet-s17f/v1/CrySTINet/data/simulated/avg_xrd_mat.npy')
def classify(self, file_name):
xrd = np.loadtxt(file_name)
xrd = torch.tensor(xrd).unsqueeze(dim=0).unsqueeze(dim=0).to(torch.float32)
# calculate cos similarity
num = np.dot(xrd.squeeze(), self.avg_xrd.T)
denom = np.linalg.norm(xrd) * np.linalg.norm(self.avg_xrd, axis=1)
similarity_res = num / denom
similarity_res = 0.5 + 0.5 * similarity_res
# test a single xrd pattern with all models
model_res = pd.DataFrame(index=self.model_kind_dict.keys(), columns=['kind', 'pred_y', 'conf'])
for idx in model_res.index:
pred_y, conf = self.eval(xrd, idx, kinds=len(self.model_kind_dict[idx]))
model_res.loc[idx] = (len(self.model_kind_dict[idx]), pred_y, conf)
# find the corresponding similarity from the similarity matrix
similarity = []
for idx in model_res.index:
pred_y_100 = self.model_kind_dict[idx][model_res.at[idx, 'pred_y']]
similarity.append(similarity_res[pred_y_100])
model_res['similarity'] = similarity
# calculate realiablity
model_res['res'] = (1-self.alpha) * model_res['conf'] + self.alpha * model_res['similarity']
return model_res
def eval(self, xrd, model, kinds):
# load trained model
# device_ids = [0, 1, 2, 3]
device_ids = [0]
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
checkpoint = torch.load(f"/bohr/crystinet-s17f/v1/CrySTINet/RCNet/checkpoints/{model}_ckpt_best.pth")
net = RCNet(n_classes=kinds)
net = net.to(device)
net = torch.nn.DataParallel(net, device_ids=device_ids)
net.load_state_dict(checkpoint['net'], strict=True)
net.eval()
out, conf = net(xrd)
pred_y = out.detach().argmax(dim=1).item()
return pred_y, conf.detach().cpu().item()
代码
文本
模型验证
代码
文本
[8]
# ----------------
# init param
# ----------------
# the trained models and structure types they contain
# the numbers are different from paper. 22~67 here are numbered 1~91 in sequence in the paper
model_kind_dict = {
# model_1
'trained_model_1': [22, 32, 55, 62, 71, 74, 82, 83, 97],
# model_2
'trained_model_2': [ 2, 6, 18, 59, 87],
# model_3
'trained_model_3': [ 1, 15, 19, 33, 36, 37, 47, 52, 53, 76, 77, 90, 96],
# model_4
'trained_model_4': [ 4, 10, 12, 30, 34, 42, 56, 60, 69, 80, 81],
# model_5
'trained_model_5': [21, 40, 41, 44, 45, 51, 64, 88, 89, 92, 94, 95, 98],
# model_6
'trained_model_6': [7, 11, 16, 29, 38, 49, 50, 63, 68, 72],
# model_7
'trained_model_7': [0, 3, 8, 13, 14, 25, 73, 85],
# model_8
'trained_model_8': [20, 27, 28, 35, 46, 54, 84],
# model_9
'trained_model_9': [ 5, 26, 39, 43, 70, 78, 79, 86, 91],
# model_10
'trained_model_10': [ 9, 17, 24, 31, 61, 67],
}
path = '/bohr/crystinet-s17f/v1/CrySTINet/data/experiment/smooth_xrd/' # XRD pattern after noise reduction
file_lst = os.listdir(path)
right_num = 0
alpha = 0.7 # the proportion of similarity
# -----------------
# init CrySTINet
# -----------------
cstrnet = CrySTINet(model_kind_dict, alpha)
# -----------------
# iterate over all single XRD pattern and test accuracy
# -----------------
for file in tqdm(file_lst):
file_name = path + file
model_res_df = cstrnet.classify(file_name)
# output
last_model = model_res_df['res'].astype(float).idxmax()
last_pred_y_100 = model_kind_dict[last_model][model_res_df.at[last_model, 'pred_y']]
label = int(file.split('@')[2].split('_')[0][4:-1])
print(f'The {file} was predicted as {last_pred_y_100} in all kinds.')
if int(last_pred_y_100) == label:
right_num += 1
print(f"The accuracy is {(right_num / len(file_lst)):.4f}")
1%|▏ | 1/80 [00:10<14:11, 10.77s/it]The RRUFF_10682_ICSD_644069@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 22 in all kinds. 2%|▎ | 2/80 [00:12<07:08, 5.50s/it]The RRUFF_1825_ICSD_160667@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 4%|▍ | 3/80 [00:14<04:52, 3.80s/it]The RRUFF_2078_ICSD_40119@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. 5%|▌ | 4/80 [00:16<03:47, 3.00s/it]The RRUFF_2192_ICSD_257588@Wurtzite-ZnS(2H)@100(40)_model_4(1)@smoothed.x was predicted as 4 in all kinds. 6%|▋ | 5/80 [00:17<03:13, 2.59s/it]The RRUFF_2257_ICSD_611033@Nickeline-NiAs@100(37)_model_2(5)@smoothed.x was predicted as 81 in all kinds. 8%|▊ | 6/80 [00:19<02:50, 2.31s/it]The RRUFF_2353_ICSD_247450@Zircon-ZrSiO4@100(64)_model_4(6)@smoothed.x was predicted as 64 in all kinds. 9%|▉ | 7/80 [00:21<02:42, 2.22s/it]The RRUFF_2428_ICSD_22411@Pyrochlore-NaCa(Nb2O6)F@100(19)_model_2(2)@smoothed.x was predicted as 19 in all kinds. 10%|█ | 8/80 [00:23<02:30, 2.09s/it]The RRUFF_2475_ICSD_193679@NaCl@100(1)_model_2(0)@smoothed.x was predicted as 5 in all kinds. 11%|█▏ | 9/80 [00:25<02:22, 2.00s/it]The RRUFF_2484_ICSD_203102@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 12%|█▎ | 10/80 [00:27<02:17, 1.96s/it]The RRUFF_2541_ICSD_75560@Corundum-Al2O3@100(69)_model_3(8)@smoothed.x was predicted as 69 in all kinds. 14%|█▍ | 11/80 [00:29<02:11, 1.90s/it]The RRUFF_2613_ICSD_71432@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 15%|█▌ | 12/80 [00:30<02:07, 1.87s/it]The RRUFF_2858_ICSD_29443@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 16%|█▋ | 13/80 [00:32<02:09, 1.94s/it]The RRUFF_2891_ICSD_94613@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 96 in all kinds. 18%|█▊ | 14/80 [00:34<02:05, 1.90s/it]The RRUFF_2993_ICSD_39173@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 19%|█▉ | 15/80 [00:36<02:01, 1.87s/it]The RRUFF_3051_ICSD_94155@Spinel-Al2MgO4@100(0)_model_6(0)@smoothed.x was predicted as 0 in all kinds. 20%|██ | 16/80 [00:38<01:58, 1.84s/it]The RRUFF_3066_ICSD_94617@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 21%|██▏ | 17/80 [00:40<01:55, 1.83s/it]The RRUFF_3072_ICSD_83460@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 22%|██▎ | 18/80 [00:42<01:57, 1.90s/it]The RRUFF_3111_ICSD_100679@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. 24%|██▍ | 19/80 [00:44<01:54, 1.88s/it]The RRUFF_3129_ICSD_41316@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 25%|██▌ | 20/80 [00:45<01:52, 1.88s/it]The RRUFF_3178_ICSD_82086@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 26%|██▋ | 21/80 [00:47<01:49, 1.86s/it]The RRUFF_3197_ICSD_100679@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. 28%|██▊ | 22/80 [00:49<01:46, 1.84s/it]The RRUFF_3251_ICSD_41316@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 2 in all kinds. 29%|██▉ | 23/80 [00:51<01:49, 1.91s/it]The RRUFF_3253_ICSD_94613@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 20 in all kinds. 30%|███ | 24/80 [00:53<01:45, 1.89s/it]The RRUFF_3306_ICSD_94617@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 31%|███▏ | 25/80 [00:55<01:42, 1.86s/it]The RRUFF_3310_ICSD_94611@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 32%|███▎ | 26/80 [00:57<01:39, 1.84s/it]The RRUFF_3335_ICSD_160667@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 34%|███▍ | 27/80 [00:58<01:37, 1.85s/it]The RRUFF_3350_ICSD_77427@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 27 in all kinds. 35%|███▌ | 28/80 [01:00<01:34, 1.82s/it]The RRUFF_3438_ICSD_40030@Spinel-Al2MgO4@100(0)_model_6(0)@smoothed.x was predicted as 0 in all kinds. 36%|███▋ | 29/80 [01:02<01:32, 1.81s/it]The RRUFF_3571_ICSD_80870@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 43 in all kinds. 38%|███▊ | 30/80 [01:04<01:32, 1.86s/it]The RRUFF_3592_ICSD_65004@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 39%|███▉ | 31/80 [01:06<01:30, 1.85s/it]The RRUFF_3602_ICSD_402574@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 40%|████ | 32/80 [01:08<01:29, 1.86s/it]The RRUFF_3628_ICSD_402574@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 41%|████▏ | 33/80 [01:09<01:26, 1.84s/it]The RRUFF_3802_ICSD_651451@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 5 in all kinds. 42%|████▎ | 34/80 [01:11<01:24, 1.85s/it]The RRUFF_3811_ICSD_41189@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 44%|████▍ | 35/80 [01:13<01:22, 1.83s/it]The RRUFF_3855_ICSD_182391@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 73 in all kinds. 45%|████▌ | 36/80 [01:15<01:23, 1.90s/it]The RRUFF_3900_ICSD_157428@Scheelite-CaWO4@100(47)_model_2(6)@smoothed.x was predicted as 61 in all kinds. 46%|████▋ | 37/80 [01:17<01:20, 1.88s/it]The RRUFF_3904_ICSD_157428@Scheelite-CaWO4@100(47)_model_2(6)@smoothed.x was predicted as 47 in all kinds. 48%|████▊ | 38/80 [01:19<01:17, 1.85s/it]The RRUFF_4054_ICSD_69644@Zircon-ZrSiO4@100(64)_model_4(6)@smoothed.x was predicted as 64 in all kinds. 49%|████▉ | 39/80 [01:21<01:15, 1.83s/it]The RRUFF_4167_ICSD_71432@Sodalite-frame@100(61)_model_9(4)@smoothed.x was predicted as 61 in all kinds. 50%|█████ | 40/80 [01:22<01:13, 1.84s/it]The RRUFF_4295_ICSD_187919@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 51%|█████▏ | 41/80 [01:24<01:13, 1.88s/it]The RRUFF_4365_ICSD_160667@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 52%|█████▎ | 42/80 [01:26<01:10, 1.86s/it]The RRUFF_4401_ICSD_651457@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 24 in all kinds. 54%|█████▍ | 43/80 [01:28<01:07, 1.83s/it]The RRUFF_4441_ICSD_18014@NaCl@100(1)_model_2(0)@smoothed.x was predicted as 1 in all kinds. 55%|█████▌ | 44/80 [01:30<01:05, 1.81s/it]The RRUFF_4592_ICSD_187193@Zircon-ZrSiO4@100(64)_model_4(6)@smoothed.x was predicted as 64 in all kinds. 56%|█████▋ | 45/80 [01:32<01:04, 1.84s/it]The RRUFF_4888_ICSD_16750@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 57%|█████▊ | 46/80 [01:34<01:04, 1.89s/it]The RRUFF_4941_ICSD_184079@Wurtzite-ZnS(2H)@100(40)_model_4(1)@smoothed.x was predicted as 40 in all kinds. 59%|█████▉ | 47/80 [01:36<01:02, 1.90s/it]The RRUFF_4953_ICSD_9582@Zircon-ZrSiO4@100(64)_model_4(6)@smoothed.x was predicted as 64 in all kinds. 60%|██████ | 48/80 [01:37<00:59, 1.86s/it]The RRUFF_5050_ICSD_51939@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 61%|██████▏ | 49/80 [01:39<00:56, 1.84s/it]The RRUFF_5065_ICSD_91380@Spinel-Al2MgO4@100(0)_model_6(0)@smoothed.x was predicted as 0 in all kinds. 62%|██████▎ | 50/80 [01:41<00:55, 1.85s/it]The RRUFF_5131_ICSD_51939@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 64%|██████▍ | 51/80 [01:43<00:53, 1.83s/it]The RRUFF_5174_ICSD_91381@Spinel-Al2MgO4@100(0)_model_6(0)@smoothed.x was predicted as 0 in all kinds. 65%|██████▌ | 52/80 [01:45<00:52, 1.88s/it]The RRUFF_540_ICSD_28827@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. 66%|██████▋ | 53/80 [01:47<00:52, 1.94s/it]The RRUFF_561_ICSD_257807@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. 68%|██████▊ | 54/80 [01:49<00:49, 1.90s/it]The RRUFF_5653_ICSD_26797@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 69%|██████▉ | 55/80 [01:50<00:46, 1.86s/it]The RRUFF_577_ICSD_252811@Scheelite-CaWO4@100(47)_model_2(6)@smoothed.x was predicted as 47 in all kinds. 70%|███████ | 56/80 [01:52<00:43, 1.83s/it]The RRUFF_5783_ICSD_92740@Zircon-ZrSiO4@100(64)_model_4(6)@smoothed.x was predicted as 64 in all kinds. 71%|███████▏ | 57/80 [01:54<00:41, 1.82s/it]The RRUFF_5981_ICSD_77576@Spinel-Al2MgO4@100(0)_model_6(0)@smoothed.x was predicted as 0 in all kinds. 72%|███████▎ | 58/80 [01:56<00:40, 1.85s/it]The RRUFF_6046_ICSD_77082@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 24 in all kinds. 74%|███████▍ | 59/80 [01:58<00:38, 1.82s/it]The RRUFF_6173_ICSD_257806@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. 75%|███████▌ | 60/80 [01:59<00:36, 1.84s/it]The RRUFF_6280_ICSD_39169@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 76%|███████▋ | 61/80 [02:01<00:34, 1.82s/it]The RRUFF_6304_ICSD_181741@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 5 in all kinds. 78%|███████▊ | 62/80 [02:03<00:33, 1.86s/it]The RRUFF_649_ICSD_108733@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 24 in all kinds. 79%|███████▉ | 63/80 [02:05<00:31, 1.88s/it]The RRUFF_6828_ICSD_77082@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 24 in all kinds. 80%|████████ | 64/80 [02:07<00:29, 1.85s/it]The RRUFF_6949_ICSD_195862@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 81%|████████▏ | 65/80 [02:09<00:27, 1.83s/it]The RRUFF_6957_ICSD_9864@NaCl@100(1)_model_2(0)@smoothed.x was predicted as 9 in all kinds. 82%|████████▎ | 66/80 [02:11<00:25, 1.84s/it]The RRUFF_6961_ICSD_43532@Sphalerite-ZnS(cF8)@100(24)_model_9(2)@smoothed.x was predicted as 26 in all kinds. 84%|████████▍ | 67/80 [02:13<00:24, 1.88s/it]The RRUFF_7069_ICSD_611040@Nickeline-NiAs@100(37)_model_2(5)@smoothed.x was predicted as 81 in all kinds. 85%|████████▌ | 68/80 [02:14<00:22, 1.85s/it]The RRUFF_7119_ICSD_90158@Corundum-Al2O3@100(69)_model_3(8)@smoothed.x was predicted as 69 in all kinds. 86%|████████▋ | 69/80 [02:16<00:20, 1.83s/it]The RRUFF_7203_ICSD_247450@Zircon-ZrSiO4@100(64)_model_4(6)@smoothed.x was predicted as 64 in all kinds. 88%|████████▊ | 70/80 [02:18<00:18, 1.82s/it]The RRUFF_745_ICSD_24945@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 89%|████████▉ | 71/80 [02:20<00:16, 1.81s/it]The RRUFF_7468_ICSD_57478@Wurtzite-ZnS(2H)@100(40)_model_4(1)@smoothed.x was predicted as 40 in all kinds. 90%|█████████ | 72/80 [02:22<00:14, 1.83s/it]The RRUFF_7473_ICSD_89034@Scheelite-CaWO4@100(47)_model_2(6)@smoothed.x was predicted as 47 in all kinds. 91%|█████████▏| 73/80 [02:23<00:12, 1.81s/it]The RRUFF_774_ICSD_51941@Rutile-TiO2@100(33)_model_2(3)@smoothed.x was predicted as 33 in all kinds. 92%|█████████▎| 74/80 [02:25<00:10, 1.83s/it]The RRUFF_8437_ICSD_15586@Scheelite-CaWO4@100(47)_model_2(6)@smoothed.x was predicted as 61 in all kinds. 94%|█████████▍| 75/80 [02:27<00:09, 1.87s/it]The RRUFF_8486_ICSD_604629@fcc(ccp)-Cu@100(9)_model_9(0)@smoothed.x was predicted as 9 in all kinds. 95%|█████████▌| 76/80 [02:29<00:07, 1.88s/it]The RRUFF_8525_ICSD_9865@NaCl@100(1)_model_2(0)@smoothed.x was predicted as 13 in all kinds. 96%|█████████▋| 77/80 [02:31<00:05, 1.86s/it]The RRUFF_8655_ICSD_24945@Garnet-Al2Ca3Si3O12@100(30)_model_3(3)@smoothed.x was predicted as 30 in all kinds. 98%|█████████▊| 78/80 [02:33<00:03, 1.83s/it]The RRUFF_8804_ICSD_44373@Pyrite-FeS2(cP12)@100(51)_model_4(5)@smoothed.x was predicted as 61 in all kinds. 99%|█████████▉| 79/80 [02:34<00:01, 1.83s/it]The RRUFF_8839_ICSD_53764@fcc(ccp)-Cu@100(9)_model_9(0)@smoothed.x was predicted as 9 in all kinds. 100%|██████████| 80/80 [02:37<00:00, 1.96s/it]The RRUFF_956_ICSD_191853@Calcite-CaCO3(hR10)@100(96)_model_2(12)@smoothed.x was predicted as 96 in all kinds. The accuracy is 0.7625
代码
文本
点个赞吧
本文被以下合集收录
机器学习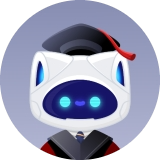
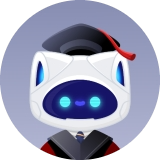
bohrb060ec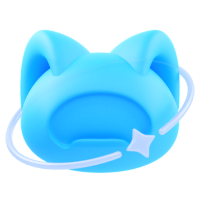
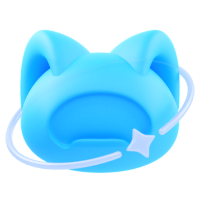
更新于 2024-07-18
17 篇0 人关注
推荐阅读
公开
基于粉末X射线衍射图谱的晶体对称性识别CNN模型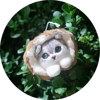
Letian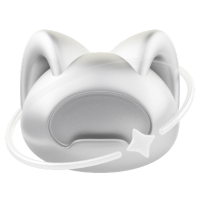
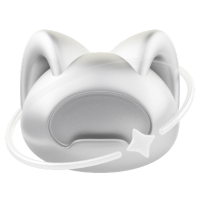
更新于 2024-06-17
2 赞2 转存文件
公开
EIS 自动化拟合等效电路模型 ECM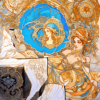
JiaweiMiao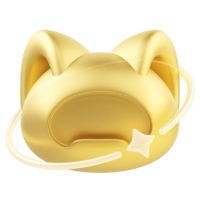
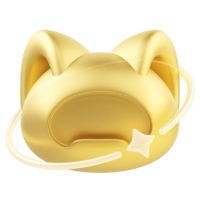
更新于 2024-07-21