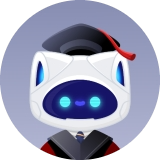
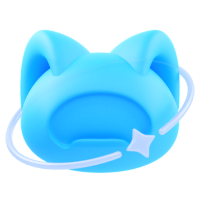
1. Introduction to Optimization Algorithms
Optimization algorithms are mathematical tools designed for finding the maximum or minimum of functions. These tools are crucial in various domains, including computational materials science, where they are used to predict material properties, optimize structures, and simulate molecular dynamics efficiently.
2. Optimization Techniques Overview
- Local Optimization: Targets finding a local maximum or minimum. Techniques include gradient descent, where the direction of steepest descent relative to the gradient is pursued.
- Global Optimization: Seeks the absolute maximum or minimum over the entire function domain. Methods like simulated annealing and genetic algorithms are notable, allowing for exploration beyond local optima.
3. Detailed Optimization Algorithms
Gradient Descent/ Steepest Descent Method: This method iteratively moves toward the minimum of a function by taking steps proportional to the negative of the gradient (or approximate gradient) of the function at the current point.
- Formula:
where:
- is the position vector after the update,
- is the current position vector,
- is the learning rate (step size),
- is the gradient of the function at .
- Formula:
where:
Conjugate Gradient Method: Particularly effective for large systems of linear equations with a positive-definite matrix. It minimizes quadratic forms directly without the need for the matrix to be stored or explicitly used.
- Key Concept: Enhances efficiency by ensuring that search directions are conjugate to each other, reducing the redundancy in search directions.
Newton's Method: Uses the second-order Taylor series expansion to find the roots of the first derivative (the zeros of the gradient), aiming for critical points where the function slope is zero.
- Formula:
For optimization, specifically finding the minimum or maximum of a function, the formula adjusts to use the gradient and Hessian:
where:
- is the Hessian matrix of second-order partial derivatives of at .
4. One-Dimensional Search Methods
These methods are pivotal when optimizing along a single direction is required, often used within broader optimization algorithms to determine optimal step sizes.
- Bracketing and Golden Section Search: Used to find a minimum within a bounded interval, relying on the property that the function decreases, then increases.
- Armijo Rule: An inexact line search method that determines a step size meeting specific sufficient decrease conditions, balancing computational cost and progress toward the minimum.
5. Application to Computational Materials Science
- Energy Minimization in Molecular Systems: Fundamental for identifying stable molecular configurations. Optimization algorithms are employed to minimize the potential energy surface, which is a high-dimensional function of all atomic positions.
- Force Field Methods: Utilize analytical functions to approximate the interactions between atoms and molecules. The Lennard-Jones potential is a classic example, offering simplicity and the ability to capture key features of molecular interactions.
Lennard-Jones Potentials Formula:
where:
- is the potential energy as a function of distance ,
- is the depth of the potential well (a measure of how strongly the two particles attract each other),
- is the finite distance at which the interparticle potential is zero (often considered the diameter of the particles),
- is the distance between the centers of the two particles.
6.1 Steepest Descent Method Implementation
6.2 Conjugate Gradient Method Implementation
6.3 Complete Python Code for Energy Minimization
This script completes the energy minimization task by:
- Defining the LJ potential and its gradient (the force).
- Generating an initial random configuration of points (representing atoms).
- Computing the total energy of the system and its gradient with respect to atomic positions.
- Implementing the steepest descent optimization method to minimize the energy by adjusting the atomic positions based on the calculated forces (gradients).
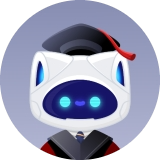
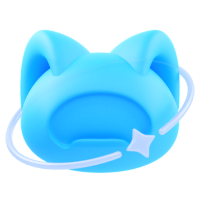
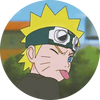
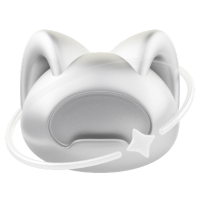