新建
Question 4 of APOAI2025 Mock Competition Baseline
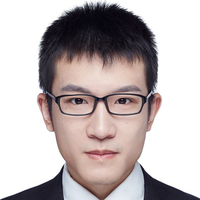
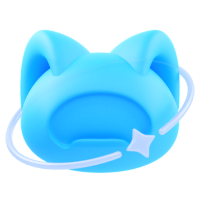
更新于 2024-12-20
推荐镜像 :ai4s-cup:0.3.1
推荐机型 :c2_m4_cpu
赞
数据集
NOAI2025教学测试第4题(v1)
[1]
import numpy as np
import torch
from torch import nn
from scipy.integrate import odeint
import pandas as pd
import matplotlib.pyplot as plt
import math
import os
def derivative(t, theta): #Use derivatives instead of finding differences.
dthetadt = (theta[1:] - theta[:-1]) / (t[1:] - t[:-1])
return dthetadt
def load_data(csv_path): #Load_data
ttheta = pd.read_csv(csv_path)
t = np.array(ttheta["t"])
theta = np.array(ttheta["theta"])
return t, theta
def split_data(t, theta, threshold = 1): #Cut down the data
theta_1 = []
t_1 = []
theta_2 = []
t_2 = []
force_flag = False
t_1.append(t[0])
theta_1.append(theta[0])
for i in range(1, len(t)):
if t[i] - t[i - 1] < threshold: #
if not force_flag:
t_1.append(t[i])
theta_1.append(theta[i])
else:
t_2.append(t[i])
theta_2.append(theta[i])
else:
force_flag = True
t_2.append(t[i])
theta_2.append(theta[i])
return np.array(t_1), np.array(theta_1), np.array(t_2), np.array(theta_2)
def theta_func(thetaomega, t, alpha, beta): #Function for solving differential equations.
theta, omega = thetaomega
a = - alpha * omega - beta * math.sin(theta)
return np.array([omega, a])
class SinglePendulum(nn.Module): #Define the equation for regression.
def __init__(self):
super().__init__()
self.alpha = nn.Parameter(torch.randn((1,), requires_grad=True))
self.beta_1 = nn.Parameter(torch.randn((1,), requires_grad=True))
self.beta_2 = nn.Parameter(torch.randn((1,), requires_grad=True))
def forward(self, theta, omega, add_force=False):
if not add_force:
a = - self.alpha * omega - self.beta_1 * torch.sin(theta)
else:
a = - self.alpha * omega - self.beta_2 * torch.sin(theta)
return a
def get_next_zerotheta_timestamp(t, theta): #To find the next zero point.
timestamp = 0
for i in range(1, len(theta)):
if theta[i - 1] * theta[i] < 0:
timestamp = t[i]
break
return timestamp
def neg_theta_func(thetaomega, t, alpha, beta): #Backward Regression
return - theta_func(thetaomega, t, alpha, beta)
def get_break_timestamp(break_t, theta_1_forward, theta_2_backward): #Find the moment when F disappears by identifying the intersection point of the curves.
min_val = math.inf
min_idx = 0
for i in range(len(break_t) - 1):
val = abs(theta_1_forward[i] - theta_2_backward[i])
dtheta_1 = theta_1_forward[i + 1] - theta_1_forward[i]
dtheta_2 = theta_2_backward[i + 1] - theta_2_backward[i]
val += abs((dtheta_1 - dtheta_2) / (break_t[i + 1] - break_t[i]))
if val < min_val:
min_val = val
min_idx = i
return break_t[min_idx]
def plot1d_full_data(t_1, theta_1, t_2, theta_2, break_t, theta_break, break_timestamp, img_path):
fig = plt.figure()
ax = fig.add_subplot()
ax.plot(t_1, theta_1, lw=1, color = 'blue')
ax.plot(t_2, theta_2, lw=1, color='blue')
ax.plot(break_t, theta_break, lw=1, color='red')
ax.scatter([break_timestamp], [theta_break[break_t == break_timestamp]], s = 20, color='red')
plt.savefig(img_path, bbox_inches="tight")
plt.show()
plt.cla()
plt.close("all")
#In this function, edit the calculation equation to require the output of the parameter,
# where csv_path is the dataset path and submission_path is the path where the generated parameters are saved.
def calculate_parameters(csv_path,submission_path):
data = {
'l': 1, # Rope length.
'miu': 1, # Air resistance.
'F': 10, # External force.
't_nextzerotheta': 1, # The moment when theta returns to zero after the next time following the application of external force and the completion of sensor observation.
't_Fput': 1 # The time of applying external force.
}
# Convert to DataFrame.
df = pd.DataFrame(data,index=[0])
# Save as a CSV file.
df.to_csv(submission_path, index=False)
print(submission_path,"is generated successfully.")
return
代码
文本
[2]
#--------Example of submission.--------#
import zipfile
import os
#Load the training set
TRAIN_PATH = "/bohr/train-08bw/v1/"
calculate_parameters(csv_path = TRAIN_PATH + "pendulum_train.csv", submission_path = "submission_train.csv") #Solve the training set equation.
submission_train.csv is generated successfully.
代码
文本
[3]
# Load the test set
#“DATA_PATH” is an environment variable for the encrypted test set.
# After submission, the test set can be accessed for system scoring in the following manner, but the participants cannot download it directly.
if os.environ.get('DATA_PATH'):
DATA_PATH = os.environ.get("DATA_PATH") + "/"
else:
print("When the baseline is running, this error message will appear because the test set cannot be read, which is a normal phenomenon.") #When the baseline is running, this error message will appear because the test set cannot be read, which is a normal phenomenon.
calculate_parameters(csv_path = DATA_PATH + "pendulum_testA.csv", submission_path = "submissionA.csv") #Solve the equation for the Public test set (test set A).
calculate_parameters(csv_path = DATA_PATH + "pendulum_testB.csv", submission_path = "submissionB.csv") #Solve the equation for the Private test set (test set B).
# Define the files to be packaged and the compressed file name.
files_to_zip = ['submissionA.csv', 'submissionB.csv']
zip_filename = 'submission.zip'
# Create a zip file.
with zipfile.ZipFile(zip_filename, 'w') as zipf:
for file in files_to_zip:
# Add files to the zip file.
zipf.write(file, os.path.basename(file))
print(f'{zip_filename} is created successfully!')
Baseline运行时,因为无法读取测试集,所以会有此条报错,属于正常现象 When the baseline is running, this error message will appear because the test set cannot be read, which is a normal phenomenon.
--------------------------------------------------------------------------- NameError Traceback (most recent call last) Input In [3], in <cell line: 9>() 7 print("Baseline运行时,因为无法读取测试集,所以会有此条报错,属于正常现象") #Baseline运行时,因为无法读取测试集,所以会有此条报错,属于正常现象 8 print("When the baseline is running, this error message will appear because the test set cannot be read, which is a normal phenomenon.") #When the baseline is running, this error message will appear because the test set cannot be read, which is a normal phenomenon. ----> 9 calculate_parameters(csv_path = DATA_PATH + "pendulum_testA.csv", submission_path = "submissionA.csv") #求解Public测试集方程,Solve the equation for the Public test set (test set A). 10 calculate_parameters(csv_path = DATA_PATH + "pendulum_testB.csv", submission_path = "submissionB.csv") #求解Private测试集方程,Solve the equation for the Private test set (test set B). 12 # 定义要打包的文件和压缩文件名, Define the files to be packaged and the compressed file name. NameError: name 'DATA_PATH' is not defined
代码
文本
点个赞吧