新建
鸢尾花书Book3_Ch05_笛卡尔坐标系__数学要素__从加减乘除到机器学习_Python_Codes
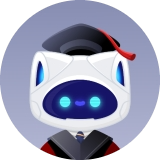
donglikun@dp.tech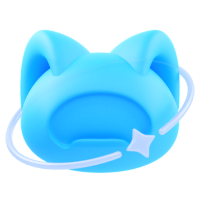
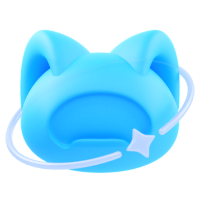
推荐镜像 :Basic Image:bohrium-notebook:2023-04-07
推荐机型 :c2_m4_cpu
赞
目录
Bk3_Ch5_01
代码
文本
[1]
# Bk3_Ch5_01
import matplotlib.pyplot as plt
import numpy as np
x = [4, 0, -5, -4, 6, 0]
y = [2, -2, 7, -6, -5, 0]
labels = ['A', 'B', 'C', 'D', 'E', 'O']
fig, ax = plt.subplots()
plt.plot(x, y, 'x')
for label, i, j in zip(labels, x, y):
plt.text(i, j+0.5, label + ' ({}, {})'.format(i, j))
plt.xlabel('x'); plt.ylabel('y')
plt.axhline(y=0, color='k', linestyle='-')
plt.axvline(x=0, color='k', linestyle='-')
plt.xticks(np.arange(-8, 8 + 1, step=1))
plt.yticks(np.arange(-8, 8 + 1, step=1))
plt.axis('scaled')
ax.set_xlim(-8,8); ax.set_ylim(-8,8)
ax.spines['top'].set_visible(False); ax.spines['bottom'].set_visible(False)
ax.spines['left'].set_visible(False); ax.spines['right'].set_visible(False)
ax.grid(linestyle='--', linewidth=0.25, color=[0.5,0.5,0.5])
代码
文本
Bk3_Ch5_02
代码
文本
[2]
# Bk3_Ch5_02
import numpy as np
import matplotlib.pyplot as plt
def fig_decor(ax):
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.hlines(y=0, xmin=-8, xmax=8, color='k')
ax.vlines(x=0, ymin=-8, ymax=8, color='k')
ax.set_xticks(np.arange(-8, 8 + 1, step=1))
ax.set_yticks(np.arange(-8, 8 + 1, step=1))
ax.axis('scaled')
ax.grid(linestyle='--', linewidth=0.25, color=[0.5,0.5,0.5])
ax.set_xbound(lower = -8, upper = 8)
ax.set_ybound(lower = -8, upper = 8)
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.spines['bottom'].set_visible(False)
ax.spines['left'].set_visible(False)
x_array = np.linspace(-8,8)
y_array = np.linspace(-8,8)
# orthogonal
fig, ax = plt.subplots()
y1 = 0.5*x_array + 2
y2 = -2*x_array - 1
ax.plot(x_array, y1)
ax.plot(x_array, y2)
fig_decor(ax)
# parallel
fig, ax = plt.subplots()
y1 = 0.5*x_array + 2
y2 = 0.5*x_array - 4
ax.plot(x_array, y1)
ax.plot(x_array, y2)
fig_decor(ax)
# horizontal
fig, ax = plt.subplots()
y1 = 0*x_array + 2
y2 = 0*x_array - 4
ax.plot(x_array, y1)
# axhline
ax.plot(x_array, y2)
fig_decor(ax)
# vertical
fig, ax = plt.subplots()
x1 = 0*y_array + 2
x2 = 0*y_array - 4
ax.plot(x1, y_array)
# axvline
ax.plot(x2, y_array)
fig_decor(ax)
代码
文本
Bk3_Ch5_03
代码
文本
[3]
# Bk3_Ch5_03
import numpy as np
import matplotlib.pyplot as plt
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
x_array = np.arange(0, 30 + 1, step=1)
y_line_1 = 35 - x_array
y_line_2 = (94 - 2*x_array)/4
fig, ax = plt.subplots()
plt.plot(x_array, y_line_1, color = '#0070C0')
plt.plot(x_array, y_line_2, color = 'g')
# solution of linear equations
plt.plot(23,12,marker = 'x', markersize = 12)
plt.axvline(x=23, color='r', linestyle='--')
plt.axhline(y=12, color='r', linestyle='--')
plt.xlabel('$x_1$ (number of chickens)')
plt.ylabel('$x_2$ (number of rabbits)')
plt.axhline(y=0, color='k', linestyle='-')
plt.axvline(x=0, color='k', linestyle='-')
plt.xticks(np.arange(0, 30 + 1, step=5))
plt.yticks(np.arange(0, 30 + 1, step=5))
plt.axis('scaled')
plt.minorticks_on()
ax.grid(which='minor', linestyle=':',
linewidth='0.5', color=[0.8, 0.8, 0.8])
ax.set_xlim(0,30); ax.set_ylim(0,30)
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.spines['bottom'].set_visible(False)
ax.spines['left'].set_visible(False)
ax.grid(linestyle='--', linewidth=0.25, color=[0.5,0.5,0.5])
代码
文本
Streamlit_Bk3_Ch5_03
代码
文本
[5]
pip install streamlit
Looking in indexes: https://pypi.tuna.tsinghua.edu.cn/simple Collecting streamlit Downloading https://pypi.tuna.tsinghua.edu.cn/packages/0e/86/69fdac2ec6852304bda08e5af5b72dfa6e74dc0b3cef0d7c1e19994388ae/streamlit-1.35.0-py2.py3-none-any.whl (8.6 MB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 8.6/8.6 MB 3.7 MB/s eta 0:00:0000:0100:01 Collecting protobuf<5,>=3.20 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/15/db/7f731524fe0e56c6b2eb57d05b55d3badd80ef7d1f1ed59db191b2fdd8ab/protobuf-4.25.3-cp37-abi3-manylinux2014_x86_64.whl (294 kB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 294.6/294.6 kB 3.1 MB/s eta 0:00:00a 0:00:01 Collecting gitpython!=3.1.19,<4,>=3.0.7 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/e9/bd/cc3a402a6439c15c3d4294333e13042b915bbeab54edc457c723931fed3f/GitPython-3.1.43-py3-none-any.whl (207 kB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 207.3/207.3 kB 3.4 MB/s eta 0:00:00a 0:00:01 Requirement already satisfied: requests<3,>=2.27 in /opt/conda/lib/python3.8/site-packages (from streamlit) (2.28.2) Requirement already satisfied: altair<6,>=4.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (4.2.2) Requirement already satisfied: pyarrow>=7.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (9.0.0) Requirement already satisfied: numpy<2,>=1.19.3 in /opt/conda/lib/python3.8/site-packages (from streamlit) (1.22.4) Requirement already satisfied: tornado<7,>=6.0.3 in /opt/conda/lib/python3.8/site-packages (from streamlit) (6.2) Requirement already satisfied: toml<2,>=0.10.1 in /opt/conda/lib/python3.8/site-packages (from streamlit) (0.10.2) Collecting pydeck<1,>=0.8.0b4 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/ab/4c/b888e6cf58bd9db9c93f40d1c6be8283ff49d88919231afe93a6bcf61626/pydeck-0.9.1-py2.py3-none-any.whl (6.9 MB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 6.9/6.9 MB 3.7 MB/s eta 0:00:0000:0100:01 Collecting watchdog>=2.1.5 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/24/01/a4034a94a5f1828eb050230e7cf13af3ac23cf763512b6afe008d3def97c/watchdog-4.0.1-py3-none-manylinux2014_x86_64.whl (83 kB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 83.0/83.0 kB 5.3 MB/s eta 0:00:00 Requirement already satisfied: pillow<11,>=7.1.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (9.4.0) Requirement already satisfied: packaging<25,>=16.8 in /opt/conda/lib/python3.8/site-packages (from streamlit) (23.0) Requirement already satisfied: pandas<3,>=1.3.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (1.5.3) Collecting blinker<2,>=1.0.0 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/bb/2a/10164ed1f31196a2f7f3799368a821765c62851ead0e630ab52b8e14b4d0/blinker-1.8.2-py3-none-any.whl (9.5 kB) Requirement already satisfied: rich<14,>=10.14.0 in /opt/conda/lib/python3.8/site-packages/rich-13.3.1-py3.8.egg (from streamlit) (13.3.1) Requirement already satisfied: click<9,>=7.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (7.1.2) Requirement already satisfied: tenacity<9,>=8.1.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (8.2.1) Requirement already satisfied: typing-extensions<5,>=4.3.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (4.5.0) Requirement already satisfied: cachetools<6,>=4.0 in /opt/conda/lib/python3.8/site-packages (from streamlit) (5.3.0) Requirement already satisfied: toolz in /opt/conda/lib/python3.8/site-packages (from altair<6,>=4.0->streamlit) (0.12.0) Requirement already satisfied: jsonschema>=3.0 in /opt/conda/lib/python3.8/site-packages (from altair<6,>=4.0->streamlit) (4.17.3) Requirement already satisfied: jinja2 in /opt/conda/lib/python3.8/site-packages (from altair<6,>=4.0->streamlit) (3.1.2) Requirement already satisfied: entrypoints in /opt/conda/lib/python3.8/site-packages (from altair<6,>=4.0->streamlit) (0.4) Collecting gitdb<5,>=4.0.1 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/fd/5b/8f0c4a5bb9fd491c277c21eff7ccae71b47d43c4446c9d0c6cff2fe8c2c4/gitdb-4.0.11-py3-none-any.whl (62 kB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 62.7/62.7 kB 2.7 MB/s eta 0:00:00 Requirement already satisfied: python-dateutil>=2.8.1 in /opt/conda/lib/python3.8/site-packages (from pandas<3,>=1.3.0->streamlit) (2.8.2) Requirement already satisfied: pytz>=2020.1 in /opt/conda/lib/python3.8/site-packages (from pandas<3,>=1.3.0->streamlit) (2022.7) Requirement already satisfied: idna<4,>=2.5 in /opt/conda/lib/python3.8/site-packages (from requests<3,>=2.27->streamlit) (3.4) Requirement already satisfied: certifi>=2017.4.17 in /opt/conda/lib/python3.8/site-packages (from requests<3,>=2.27->streamlit) (2022.12.7) Requirement already satisfied: urllib3<1.27,>=1.21.1 in /opt/conda/lib/python3.8/site-packages (from requests<3,>=2.27->streamlit) (1.26.14) Requirement already satisfied: charset-normalizer<4,>=2 in /opt/conda/lib/python3.8/site-packages (from requests<3,>=2.27->streamlit) (3.0.1) Requirement already satisfied: markdown-it-py<3.0.0,>=2.1.0 in /opt/conda/lib/python3.8/site-packages/markdown_it_py-2.2.0-py3.8.egg (from rich<14,>=10.14.0->streamlit) (2.2.0) Collecting pygments<3.0.0,>=2.14.0 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/f7/3f/01c8b82017c199075f8f788d0d906b9ffbbc5a47dc9918a945e13d5a2bda/pygments-2.18.0-py3-none-any.whl (1.2 MB) ━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 1.2/1.2 MB 3.2 MB/s eta 0:00:00a 0:00:01 Collecting smmap<6,>=3.0.1 Downloading https://pypi.tuna.tsinghua.edu.cn/packages/a7/a5/10f97f73544edcdef54409f1d839f6049a0d79df68adbc1ceb24d1aaca42/smmap-5.0.1-py3-none-any.whl (24 kB) Requirement already satisfied: MarkupSafe>=2.0 in /opt/conda/lib/python3.8/site-packages (from jinja2->altair<6,>=4.0->streamlit) (2.1.1) Requirement already satisfied: importlib-resources>=1.4.0 in /opt/conda/lib/python3.8/site-packages (from jsonschema>=3.0->altair<6,>=4.0->streamlit) (5.2.0) Requirement already satisfied: pkgutil-resolve-name>=1.3.10 in /opt/conda/lib/python3.8/site-packages (from jsonschema>=3.0->altair<6,>=4.0->streamlit) (1.3.10) Requirement already satisfied: pyrsistent!=0.17.0,!=0.17.1,!=0.17.2,>=0.14.0 in /opt/conda/lib/python3.8/site-packages (from jsonschema>=3.0->altair<6,>=4.0->streamlit) (0.18.0) Requirement already satisfied: attrs>=17.4.0 in /opt/conda/lib/python3.8/site-packages (from jsonschema>=3.0->altair<6,>=4.0->streamlit) (22.1.0) Requirement already satisfied: mdurl~=0.1 in /opt/conda/lib/python3.8/site-packages/mdurl-0.1.2-py3.8.egg (from markdown-it-py<3.0.0,>=2.1.0->rich<14,>=10.14.0->streamlit) (0.1.2) Requirement already satisfied: six>=1.5 in /opt/conda/lib/python3.8/site-packages (from python-dateutil>=2.8.1->pandas<3,>=1.3.0->streamlit) (1.16.0) Requirement already satisfied: zipp>=3.1.0 in /opt/conda/lib/python3.8/site-packages (from importlib-resources>=1.4.0->jsonschema>=3.0->altair<6,>=4.0->streamlit) (3.14.0) Installing collected packages: watchdog, smmap, pygments, protobuf, blinker, pydeck, gitdb, gitpython, streamlit Attempting uninstall: pygments Found existing installation: Pygments 2.11.2 Uninstalling Pygments-2.11.2: Successfully uninstalled Pygments-2.11.2 Attempting uninstall: protobuf Found existing installation: protobuf 3.19.6 Uninstalling protobuf-3.19.6: Successfully uninstalled protobuf-3.19.6 ERROR: pip's dependency resolver does not currently take into account all the packages that are installed. This behaviour is the source of the following dependency conflicts. tensorflow 2.11.0 requires protobuf<3.20,>=3.9.2, but you have protobuf 4.25.3 which is incompatible. tensorflow-metadata 1.12.0 requires protobuf<4,>=3.13, but you have protobuf 4.25.3 which is incompatible. tensorboard 2.11.2 requires protobuf<4,>=3.9.2, but you have protobuf 4.25.3 which is incompatible. tensorboardx 2.6 requires protobuf<4,>=3.8.0, but you have protobuf 4.25.3 which is incompatible. Successfully installed blinker-1.8.2 gitdb-4.0.11 gitpython-3.1.43 protobuf-4.25.3 pydeck-0.9.1 pygments-2.18.0 smmap-5.0.1 streamlit-1.35.0 watchdog-4.0.1 WARNING: Running pip as the 'root' user can result in broken permissions and conflicting behaviour with the system package manager. It is recommended to use a virtual environment instead: https://pip.pypa.io/warnings/venv Note: you may need to restart the kernel to use updated packages.
代码
文本
[6]
import numpy as np
import matplotlib.pyplot as plt
import streamlit as st
#%%
with st.sidebar:
st.latex('y = ax + b')
a = st.slider('Slope, a:',
min_value = -4.0,
max_value = 4.0,
step = 0.1)
b = st.slider('Intercept, b:',
min_value = -4.0,
max_value = 4.0,
step = 0.1)
#%%
st.latex("y = %.2f x + %.2f" % (a, b))
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
x_array = np.arange(-10, 10 + 1, step=1)
y_array = a*x_array + b
fig, ax = plt.subplots()
plt.plot(x_array, y_array)
plt.xlabel('$x_1$')
plt.ylabel('$x_2$ ')
plt.axhline(y=0, color='k', linestyle='-')
plt.axvline(x=0, color='k', linestyle='-')
plt.xticks(np.arange(-10, 10 + 1, step=2))
plt.yticks(np.arange(-10, 10 + 1, step=2))
plt.axis('scaled')
plt.minorticks_on()
ax.grid(which='major', linestyle=':',
linewidth='0.5', color=[0.8, 0.8, 0.8])
ax.set_xlim(-10,10);
ax.set_ylim(-10,10)
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.spines['bottom'].set_visible(False)
ax.spines['left'].set_visible(False)
ax.grid(linestyle='--', linewidth=0.25, color=[0.5,0.5,0.5])
st.pyplot(fig)
2024-06-03 16:23:30.129
Warning: to view this Streamlit app on a browser, run it with the following
command:
streamlit run /opt/conda/lib/python3.8/site-packages/ipykernel_launcher.py [ARGUMENTS]
2024-06-03 16:23:30.130 Session state does not function when running a script without `streamlit run`
DeltaGenerator()
代码
文本
Bk3_Ch5_04
代码
文本
[7]
# Bk3_Ch5_04
import numpy as np
import matplotlib.pyplot as plt
def plot_polar(theta, r):
fig, ax = plt.subplots(subplot_kw={'projection': 'polar'})
ax.plot(theta, r)
# set radial axis limit
ax.set_rmax(20)
# set radial axis ticks
ax.set_rticks([5, 10, 15, 20])
# position radial labels
ax.set_rlabel_position(-45)
ax.set_thetagrids(np.arange(0.0, 360.0, 45.0));
plt.show()
#%% circle
theta = np.linspace(0, 6*np.pi, 1000)
r = 10 + theta*0
plot_polar(theta, r)
#%% Archimedes' spiral
r = 1*theta
plot_polar(theta, r)
#%% Rose
r = 10*np.cos(6*theta) + 10
plot_polar(theta, r)
代码
文本
Bk3_Ch5_05
代码
文本
[ ]
# Bk3_Ch5_05
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0,2*np.pi,100)
# parametric equation of unit circle
x1 = np.cos(t)
x2 = np.sin(t)
fig, ax = plt.subplots()
# plot the circle
plt.plot(x1,x2)
plt.show()
ax.set_xlabel('$x_1$'); ax.set_ylabel('$x_2$')
ax.set_xticks(np.arange(-2, 2 + 1, step=1))
ax.set_yticks(np.arange(-2, 2 + 1, step=1))
ax.axis('scaled')
ax.grid(linestyle='--', linewidth=0.25, color=[0.7,0.7,0.7])
ax.set_xbound(lower = -2, upper = 2); ax.set_ybound(lower = -2, upper = 2)
plt.gca().spines['right'].set_visible(False)
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['left'].set_visible(False)
plt.gca().spines['bottom'].set_visible(False)
plt.axhline(y=0, color='k', linestyle='-')
plt.axvline(x=0, color='k', linestyle='-')
代码
文本
Bk3_Ch5_06
代码
文本
[ ]
# Bk3_Ch5_06
from sympy import *
from sympy.plotting import plot_parametric
import math
t = symbols('t')
# parametric equation of unit circle
x1 = cos(t)
x2 = sin(t)
# plot the circle
plot_parametric(x1, x2, (t, 0, 2*pi), size = (10,10))
代码
文本
点个赞吧
本文被以下合集收录
鸢尾花书Book3——数学要素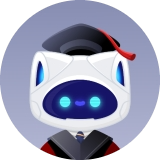
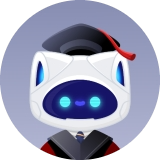
donglikun@dp.tech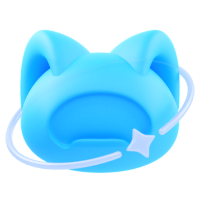
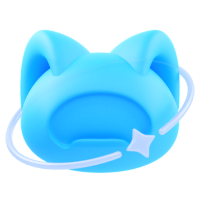
更新于 2024-05-29
25 篇0 人关注
推荐阅读
公开
鸢尾花书Book3_Ch24_鸡兔同笼2__数学要素__从加减乘除到机器学习_Python_Codes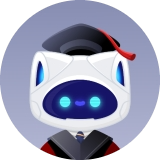
donglikun@dp.tech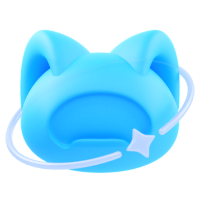
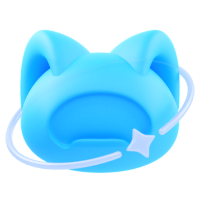
发布于 2024-05-30
公开
鸢尾花书Book3_Ch07_距离__数学要素__从加减乘除到机器学习_Python_Codes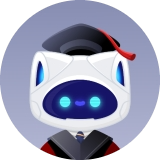
donglikun@dp.tech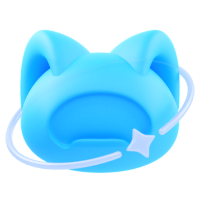
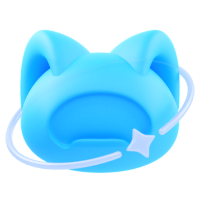
更新于 2024-06-06